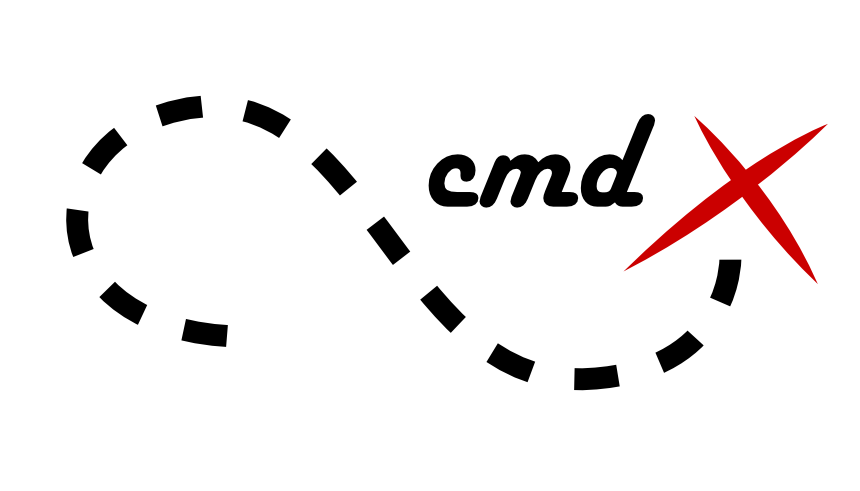
The fast subset of `maya.cmds`
cmdx¶
cmdx is a Python module for fast scenegraph manipulation in Autodesk Maya.
- Documentation
- Command reference below
API¶
The basics from maya.cmds are included.
-
cmdx.
createNode
(type, name=None, parent=None)¶ Create a new node
This function forms the basic building block with which to create new nodes in Maya.
Note
Missing arguments shared and skipSelect
Tip
For additional performance, type may be given as an MTypeId
Parameters: - type (str) – Type name of new node, e.g. “transform”
- name (str, optional) – Sets the name of the newly-created node
- parent (Node, optional) – Specifies the parent in the DAG under which the new node belongs
Example
>>> node = createNode("transform") # Type as string >>> node = createNode(tTransform) # Type as ID
# Shape without transform automatically creates transform >>> node = createNode(“mesh”) >>> node.isA(kTransform) True
# DG nodes are also OK >>> node = createNode(“multMatrix”)
# Parenting is A-OK >>> parent = createNode(“transform”) >>> child = createNode(“transform”, parent=parent) >>> child.parent() == parent True
# Custom name is OK >>> specialName = createNode(“transform”, name=”specialName”) >>> specialName.name() == “specialName” True
-
cmdx.
getAttr
(attr, type=None, time=None)¶ Read attr
Parameters: - attr (Plug) – Attribute as a cmdx.Plug
- type (str, optional) – Unused
- time (float, optional) – Time at which to evaluate the attribute
Example
>>> node = createNode("transform") >>> getAttr(node + ".translateX") 0.0
-
cmdx.
setAttr
(attr, value, type=None)¶ Write value to attr
Parameters: - attr (Plug) – Existing attribute to edit
- value (any) – Value to write
- type (int, optional) – Unused
Example
>>> node = createNode("transform") >>> setAttr(node + ".translateX", 5.0)
-
cmdx.
addAttr
(node, longName, attributeType, shortName=None, enumName=None, defaultValue=None)¶ Add new attribute to node
Parameters: - node (Node) – Add attribute to this node
- longName (str) – Name of resulting attribute
- attributeType (str) – Type of attribute, e.g. string
- shortName (str, optional) – Alternate name of attribute
- enumName (str, optional) – Options for an enum attribute
- defaultValue (any, optional) – Default value of attribute
Example
>>> node = createNode("transform") >>> addAttr(node, "myString", attributeType="string") >>> addAttr(node, "myDouble", attributeType=Double)
-
cmdx.
connectAttr
(src, dst)¶ Connect src to dst
Parameters: Example
>>> src = createNode("transform") >>> dst = createNode("transform") >>> connectAttr(src + ".rotateX", dst + ".scaleY")
-
cmdx.
listRelatives
(node, type=None, children=False, allDescendents=False, parent=False, shapes=False)¶ List relatives of node
Parameters: - node (DagNode) – Node to enquire about
- type (int, optional) – Only return nodes of this type
- children (bool, optional) – Return children of node
- parent (bool, optional) – Return parent of node
- shapes (bool, optional) – Return only children that are shapes
- allDescendents (bool, optional) – Return descendents of node
- fullPath (bool, optional) – Unused; nodes are always exact
- path (bool, optional) – Unused; nodes are always exact
Example
>>> parent = createNode("transform") >>> child = createNode("transform", parent=parent) >>> listRelatives(child, parent=True) == [parent] True >>> listRelatives(str(child), parent=True) == [str(parent)] True
-
cmdx.
listConnections
(attr)¶ List connections of attr
Parameters: attr (Plug or Node) – Example
>>> node1 = createNode("transform") >>> node2 = createNode("mesh", parent=node1) >>> node1["v"] >> node2["v"] >>> listConnections(node1) == [node2] True >>> listConnections(node1 + ".v") == [node2] True >>> listConnections(node1["v"]) == [node2] True >>> listConnections(node2) == [node1] True
Object Oriented Interface¶
In addition to the familiar interface inherited from cmds, cmdx offers a faster, object oriented alternative to all of that functionality, and more.
-
class
cmdx.
Node
(mobject, exists=True)¶ A Maya dependency node
Example
>>> _ = cmds.file(new=True, force=True) >>> decompose = createNode("decomposeMatrix", name="decompose") >>> str(decompose) 'decompose' >>> alias = encode(decompose.name()) >>> decompose == alias True >>> transform = createNode("transform") >>> transform["tx"] = 5 >>> transform["worldMatrix"][0] >> decompose["inputMatrix"] >>> decompose["outputTranslate"] (5.0, 0.0, 0.0)
-
addAttr
(attr)¶ Add a new dynamic attribute to node
Parameters: attr (Plug) – Add this attribute Example
>>> node = createNode("transform") >>> attr = Double("myAttr", default=5.0) >>> node.addAttr(attr) >>> node["myAttr"] == 5.0 True
-
add_attr
(attr)¶ Add a new dynamic attribute to node
Parameters: attr (Plug) – Add this attribute Example
>>> node = createNode("transform") >>> attr = Double("myAttr", default=5.0) >>> node.addAttr(attr) >>> node["myAttr"] == 5.0 True
-
clear
()¶ Clear transient state
A node may cache previously queried values for performance at the expense of memory. This method erases any cached values, freeing up memory at the expense of performance.
Example
>>> node = createNode("transform") >>> node["translateX"] = 5 >>> node["translateX"] 5.0 >>> # Plug was reused >>> node["translateX"] 5.0 >>> # Value was reused >>> node.clear() >>> node["translateX"] 5.0 >>> # Plug and value was recomputed
-
code
¶ Return MObjectHandle.hashCode of this node
This a guaranteed-unique integer (long in Python 2) similar to the UUID of Maya 2016
-
connection
(type=None, unit=None, plug=False, source=True, destination=True, connection=False)¶ Singular version of
connections()
-
connections
(type=None, unit=None, plugs=False, source=True, destination=True, connections=False)¶ Yield plugs of node with a connection to any other plug
Parameters: - unit (int, optional) – Return plug in this unit, e.g. Meters or Radians
- type (str, optional) – Restrict output to nodes of this type, e.g. “transform” or “mesh”
- plugs (bool, optional) – Return plugs, rather than nodes
- source (bool, optional) – Return inputs only
- destination (bool, optional) – Return outputs only
- connections (bool, optional) – Return tuples of the connected plugs
Example
>>> _ = cmds.file(new=True, force=True) >>> a = createNode("transform", name="A") >>> b = createNode("multDoubleLinear", name="B") >>> a["ihi"] << b["ihi"] >>> list(a.connections()) == [b] True >>> list(b.connections()) == [a] True >>> a.connection() == b True
-
data
¶ Special handling for data stored in the instance
Normally, the initialisation of data could happen in the __init__, but for some reason the postConstructor of a custom plug-in calls __init__ twice for every unique hex, which causes any data added there to be wiped out once the postConstructor is done.
-
deleteAttr
(attr)¶ Delete attr from node
Parameters: attr (Plug) – Attribute to remove Example
>>> node = createNode("transform") >>> node["myAttr"] = Double() >>> node.deleteAttr("myAttr") >>> node.hasAttr("myAttr") False
-
delete_attr
(attr)¶ Delete attr from node
Parameters: attr (Plug) – Attribute to remove Example
>>> node = createNode("transform") >>> node["myAttr"] = Double() >>> node.deleteAttr("myAttr") >>> node.hasAttr("myAttr") False
-
destroyed
¶
-
dump
(ignore_error=True, preserve_order=False)¶ Return dictionary of all attributes
Example
>>> import json >>> _ = cmds.file(new=True, force=True) >>> node = createNode("choice") >>> dump = node.dump() >>> isinstance(dump, dict) True >>> dump["choice1.caching"] False
-
dumps
(indent=4, sort_keys=True, preserve_order=False)¶ Return a JSON compatible dictionary of all attributes
-
exists
¶ The node exists in both memory and scene
Example
>>> node = createNode("joint") >>> node.exists True >>> cmds.delete(str(node)) >>> node.exists False >>> _ = cmds.file(new=True, force=True) >>> node.exists False
-
findPlug
(name, cached=False, safe=True)¶ Cache previously found plugs, for performance
Cost: 4.9 microseconds/call
Part of the time taken in querying an attribute is the act of finding a plug given its name as a string.
This causes a 25% reduction in time taken for repeated attribute queries. Though keep in mind that state is stored in the cmdx object which currently does not survive rediscovery. That is, if a node is created and later discovered through a call to encode, then the original and discovered nodes carry one state each.
Additional challenges include storing the same plug for both long and short name of said attribute, which is currently not the case.
Parameters: - name (str) – Name of plug to find
- cached (bool, optional) – (DEPRECATED) Return cached plug, or throw an exception. Default to False, which means it will run Maya’s findPlug() and cache the result.
- safe (bool, optional) – (DEPRECATED) Always find the plug through Maya’s API, defaults to True. This will not perform any caching and is intended for use during debugging to spot whether caching is causing trouble.
Example
>>> node = createNode("transform") >>> plug1 = node.findPlug("translateX") >>> isinstance(plug1, om.MPlug) True >>> plug1 == node.findPlug("translateX") True
-
find_plug
(name, cached=False, safe=True)¶ Cache previously found plugs, for performance
Cost: 4.9 microseconds/call
Part of the time taken in querying an attribute is the act of finding a plug given its name as a string.
This causes a 25% reduction in time taken for repeated attribute queries. Though keep in mind that state is stored in the cmdx object which currently does not survive rediscovery. That is, if a node is created and later discovered through a call to encode, then the original and discovered nodes carry one state each.
Additional challenges include storing the same plug for both long and short name of said attribute, which is currently not the case.
Parameters: - name (str) – Name of plug to find
- cached (bool, optional) – (DEPRECATED) Return cached plug, or throw an exception. Default to False, which means it will run Maya’s findPlug() and cache the result.
- safe (bool, optional) – (DEPRECATED) Always find the plug through Maya’s API, defaults to True. This will not perform any caching and is intended for use during debugging to spot whether caching is causing trouble.
Example
>>> node = createNode("transform") >>> plug1 = node.findPlug("translateX") >>> isinstance(plug1, om.MPlug) True >>> plug1 == node.findPlug("translateX") True
-
hasAttr
(attr)¶ Return whether or not attr exists
Parameters: attr (str) – Name of attribute to check Example
>>> node = createNode("transform") >>> node.hasAttr("mysteryAttribute") False >>> node.hasAttr("translateX") True >>> node["myAttr"] = Double() # Dynamic attribute >>> node.hasAttr("myAttr") True
-
has_attr
(attr)¶ Return whether or not attr exists
Parameters: attr (str) – Name of attribute to check Example
>>> node = createNode("transform") >>> node.hasAttr("mysteryAttribute") False >>> node.hasAttr("translateX") True >>> node["myAttr"] = Double() # Dynamic attribute >>> node.hasAttr("myAttr") True
-
hashCode
¶ Return MObjectHandle.hashCode of this node
This a guaranteed-unique integer (long in Python 2) similar to the UUID of Maya 2016
-
hash_code
¶ Return MObjectHandle.hashCode of this node
This a guaranteed-unique integer (long in Python 2) similar to the UUID of Maya 2016
-
hex
¶ Return unique hashCode as hexadecimal string
Example
>>> node = createNode("transform") >>> node.hexStr == format(node.hashCode, "x") True
-
hexStr
¶ Return unique hashCode as hexadecimal string
Example
>>> node = createNode("transform") >>> node.hexStr == format(node.hashCode, "x") True
-
hex_str
¶ Return unique hashCode as hexadecimal string
Example
>>> node = createNode("transform") >>> node.hexStr == format(node.hashCode, "x") True
-
index
(plug)¶ - Find index of attr in its owning node
# TODO: This is really slow
-
input
(type=None, unit=None, plug=None, connection=False)¶ Return first input connection from
connections()
-
inputs
(type=None, unit=None, plugs=False, connections=False)¶ Return input connections from
connections()
-
instance
()¶ Return the current plug-in instance of this node
-
isA
(type)¶ Evaluate whether self is of type
Parameters: type (MTypeId, str, list, int) – any kind of type to check - MFn function set constant - MTypeId objects - nodetype names Example
>>> node = createNode("transform") >>> node.isA(kTransform) True >>> node.isA(kShape) False >>> node.isA((kShape, kTransform)) True
-
isAlive
()¶ The node exists somewhere in memory
-
isLocked
()¶
-
isReferenced
()¶
-
is_a
(type)¶ Evaluate whether self is of type
Parameters: type (MTypeId, str, list, int) – any kind of type to check - MFn function set constant - MTypeId objects - nodetype names Example
>>> node = createNode("transform") >>> node.isA(kTransform) True >>> node.isA(kShape) False >>> node.isA((kShape, kTransform)) True
-
is_alive
()¶ The node exists somewhere in memory
-
is_locked
()¶
-
is_referenced
()¶
-
lock
(value=True)¶
-
name
(namespace=False)¶ Return the name of this node
Parameters: namespace (bool, optional) – Return with namespace, defaults to False Example
>>> node = createNode("transform", name="myName") >>> node.name() == 'myName' True
-
namespace
()¶ Get namespace of node
Example
>>> _ = cmds.file(new=True, force=True) >>> node = createNode("transform", name="myNode") >>> node.namespace() == "" True >>> _ = cmds.namespace(add=":A") >>> _ = cmds.namespace(add=":A:B") >>> node = createNode("transform", name=":A:B:myNode") >>> node.namespace() == 'A:B' True
-
object
()¶ Return MObject of this node
-
output
(type=None, plug=False, unit=None, connection=False)¶ Return first output connection from
connections()
-
outputs
(type=None, plugs=False, unit=None, connections=False)¶ Return output connections from
connections()
-
path
()¶
-
plugin
()¶ Return the user-defined class of the plug-in behind this node
-
pop
(key)¶ Delete an attribute
Parameters: key (str) – Name of attribute to delete Example
>>> node = createNode("transform") >>> node["myAttr"] = Double() >>> node.pop("myAttr") >>> node.hasAttr("myAttr") False
-
removed
¶
-
rename
(name)¶
-
shortestPath
()¶
-
shortest_path
()¶
-
storable
¶ Whether or not to save this node with the file
-
type
()¶ Return type name
Example
>>> node = createNode("choice") >>> node.type() == 'choice' True
-
typeId
¶ Return the native maya.api.MTypeId of this node
Example
>>> node = createNode("transform") >>> node.typeId == tTransform True
-
typeName
¶
-
type_id
¶ Return the native maya.api.MTypeId of this node
Example
>>> node = createNode("transform") >>> node.typeId == tTransform True
-
type_name
¶
-
update
(attrs)¶ Apply a series of attributes all at once
This operates similar to a Python dictionary.
Parameters: attrs (dict) – Key/value pairs of name and attribute Examples
>>> node = createNode("transform") >>> node.update({"tx": 5.0, ("ry", Degrees): 30.0}) >>> node["tx"] 5.0
-
-
class
cmdx.
DagNode
(mobject, exists=True)¶ A Maya DAG node
The difference between this and Node is that a DagNode can have one or more children and one parent (multiple parents not supported).
Example
>>> _ = cmds.file(new=True, force=True) >>> parent = createNode("transform") >>> child = createNode("transform", parent=parent) >>> child.parent() == parent True >>> next(parent.children()) == child True >>> parent.child() == child True >>> sibling = createNode("transform", parent=parent) >>> child.sibling() == sibling True >>> shape = createNode("mesh", parent=child) >>> child.shape() == shape True >>> shape.parent() == child True
-
addChild
(child, index=-1, safe=True)¶ Add child to self
Parameters: - child (Node) – Child to add
- index (int, optional) – Physical location in hierarchy, defaults to cmdx.Last
- safe (bool) – Prevents crash when the node to reparent was formerly a descendent of the new parent. Costs 6µs/call
Example
>>> parent = createNode("transform") >>> child = createNode("transform") >>> parent.addChild(child)
-
add_child
(child, index=-1, safe=True)¶ Add child to self
Parameters: - child (Node) – Child to add
- index (int, optional) – Physical location in hierarchy, defaults to cmdx.Last
- safe (bool) – Prevents crash when the node to reparent was formerly a descendent of the new parent. Costs 6µs/call
Example
>>> parent = createNode("transform") >>> child = createNode("transform") >>> parent.addChild(child)
-
assembly
()¶ Return the top-level parent of node
Example
>>> parent1 = createNode("transform") >>> parent2 = createNode("transform") >>> child = createNode("transform", parent=parent1) >>> grandchild = createNode("transform", parent=child) >>> child.assembly() == parent1 True >>> parent2.assembly() == parent2 True
-
boundingBox
¶ Return a cmdx.BoundingBox of this DAG node
-
bounding_box
¶ Return a cmdx.BoundingBox of this DAG node
-
child
(type=None, filter=110, query=None, contains=None)¶
-
childCount
(type=None)¶ Return number of children of a given optional type
Compared to MFnDagNode.childCount, this function actually returns children, not shapes, along with filtering by an optional type.
Parameters: type (str) – Same as to .children(type=)
-
child_count
(type=None)¶ Return number of children of a given optional type
Compared to MFnDagNode.childCount, this function actually returns children, not shapes, along with filtering by an optional type.
Parameters: type (str) – Same as to .children(type=)
-
children
(type=None, filter=110, query=None, contains=None)¶ Return children of node
All returned children are transform nodes, as specified by the filter argument. For shapes, use the
shapes()
method. The contains argument only returns transform nodes containing a shape of the type provided.Parameters: - type (str, optional) – Return only children that match this type
- filter (int, optional) – Return only children with this function set
- contains (str, optional) – Child must have a shape of this type
- query (dict, optional) – Limit output to nodes with these attributes
Example
>>> _ = cmds.file(new=True, force=True) >>> a = createNode("transform", "a") >>> b = createNode("transform", "b", parent=a) >>> c = createNode("transform", "c", parent=a) >>> d = createNode("mesh", "d", parent=c) >>> list(a.children()) == [b, c] True >>> a.child() == b True >>> c.child(type="mesh") >>> c.child(type="mesh", filter=None) == d True >>> c.child(type=("mesh", "transform"), filter=None) == d True >>> a.child() == b True >>> a.child(contains="mesh") == c True >>> a.child(contains="nurbsCurve") is None True >>> b["myAttr"] = Double(default=5) >>> a.child(query=["myAttr"]) == b True >>> a.child(query=["noExist"]) is None True >>> a.child(query={"myAttr": 5}) == b True >>> a.child(query={"myAttr": 1}) is None True
-
clone
(name=None, parent=None, worldspace=False)¶ Return a clone of self
A “clone” assignes the .outMesh attribute of a mesh node to the .inMesh of the resulting clone.
- Supports:
- mesh
Parameters: - name (str, optional) – Name of newly created clone
- parent (DagNode, optional) – Parent to newly cloned node
- worldspace (bool, optional) – Translate output to worldspace
-
dagPath
()¶ Return a om.MDagPath for this node
Example
>>> _ = cmds.file(new=True, force=True) >>> parent = createNode("transform", name="Parent") >>> child = createNode("transform", name="Child", parent=parent) >>> path = child.dagPath() >>> str(path) 'Child' >>> str(path.pop()) 'Parent'
-
dag_path
()¶ Return a om.MDagPath for this node
Example
>>> _ = cmds.file(new=True, force=True) >>> parent = createNode("transform", name="Parent") >>> child = createNode("transform", name="Child", parent=parent) >>> path = child.dagPath() >>> str(path) 'Child' >>> str(path.pop()) 'Parent'
-
descendent
(type=0)¶ Singular version of
descendents()
A recursive, depth-first search.
a | b---d | | c e
Example
>>> _ = cmds.file(new=True, force=True) >>> a = createNode("transform", "a") >>> b = createNode("transform", "b", parent=a) >>> c = createNode("transform", "c", parent=b) >>> d = createNode("transform", "d", parent=b) >>> e = createNode("transform", "e", parent=d) >>> a.descendent() == a.child() True >>> list(a.descendents()) == [b, c, d, e] True >>> f = createNode("mesh", "f", parent=e) >>> list(a.descendents(type="mesh")) == [f] True
-
descendents
(type=None)¶ Faster and more efficient dependency graph traversal
Requires Maya 2017+
Example
>>> grandparent = createNode("transform") >>> parent = createNode("transform", parent=grandparent) >>> child = createNode("transform", parent=parent) >>> mesh = createNode("mesh", parent=child) >>> it = grandparent.descendents(type=tMesh) >>> next(it) == mesh True >>> next(it) Traceback (most recent call last): ... StopIteration
-
duplicate
()¶ Return a duplicate of self
-
enableLimit
(typ, state)¶
-
enable_limit
(typ, state)¶
-
hide
()¶ Set visibility to False
-
isLimited
(typ)¶
-
is_limited
(typ)¶
-
level
¶ Return the number of parents this DAG node has
Example
>>> parent = createNode("transform") >>> child = createNode("transform", parent=parent) >>> child.level 1 >>> parent.level 0
-
limitValue
(typ)¶
-
limit_value
(typ)¶
-
lineage
(type=None, filter=None)¶ Yield parents all the way up a hierarchy
Example
>>> _ = cmds.file(new=True, force=True) >>> a = createNode("transform", name="a") >>> b = createNode("transform", name="b", parent=a) >>> c = createNode("transform", name="c", parent=b) >>> d = createNode("transform", name="d", parent=c) >>> lineage = d.lineage() >>> next(lineage) == c True >>> next(lineage) == b True >>> next(lineage) == a True
-
mapFrom
(other, time=None)¶ Return TransformationMatrix of other relative self
Example
>>> a = createNode("transform") >>> b = createNode("transform") >>> a["translate"] = (0, 5, 0) >>> b["translate"] = (0, -5, 0) >>> delta = a.mapFrom(b) >>> delta.translation()[1] 10.0 >>> a = createNode("transform") >>> b = createNode("transform") >>> a["translate"] = (0, 5, 0) >>> b["translate"] = (0, -15, 0) >>> delta = a.mapFrom(b) >>> delta.translation()[1] 20.0
-
mapTo
(other, time=None)¶ Return TransformationMatrix of self relative other
See
mapFrom()
for examples.
-
map_from
(other, time=None)¶ Return TransformationMatrix of other relative self
Example
>>> a = createNode("transform") >>> b = createNode("transform") >>> a["translate"] = (0, 5, 0) >>> b["translate"] = (0, -5, 0) >>> delta = a.mapFrom(b) >>> delta.translation()[1] 10.0 >>> a = createNode("transform") >>> b = createNode("transform") >>> a["translate"] = (0, 5, 0) >>> b["translate"] = (0, -15, 0) >>> delta = a.mapFrom(b) >>> delta.translation()[1] 20.0
-
map_to
(other, time=None)¶ Return TransformationMatrix of self relative other
See
mapFrom()
for examples.
-
parent
(type=None, filter=None)¶ Return parent of node
Parameters: type (str, optional) – Return parent, only if it matches this type Example
>>> parent = createNode("transform") >>> child = createNode("transform", parent=parent) >>> child.parent() == parent True >>> not child.parent(type="camera") True >>> parent.parent() >>> child.parent(filter=om.MFn.kTransform) == parent True >>> child.parent(filter=om.MFn.kJoint) is None True
Returns: If any, else None Return type: parent (Node)
-
parenthood
(type=None, filter=None)¶ Yield parents all the way up a hierarchy
Example
>>> _ = cmds.file(new=True, force=True) >>> a = createNode("transform", name="a") >>> b = createNode("transform", name="b", parent=a) >>> c = createNode("transform", name="c", parent=b) >>> d = createNode("transform", name="d", parent=c) >>> lineage = d.lineage() >>> next(lineage) == c True >>> next(lineage) == b True >>> next(lineage) == a True
-
path
()¶ Return full path to node
Example
>>> parent = createNode("transform", "myParent") >>> child = createNode("transform", "myChild", parent=parent) >>> child.name() == 'myChild' True >>> child.path() == '|myParent|myChild' True
-
root
()¶ Return the top-level parent of node
Example
>>> parent1 = createNode("transform") >>> parent2 = createNode("transform") >>> child = createNode("transform", parent=parent1) >>> grandchild = createNode("transform", parent=child) >>> child.assembly() == parent1 True >>> parent2.assembly() == parent2 True
-
rotation
(space=2, time=None)¶ Convenience method for transform(space).rotation()
Example
>>> node = createNode("transform") >>> node["rotateX"] = radians(90) >>> round(degrees(node.rotation().x), 1) 90.0
-
scale
(space=2, time=None)¶ Convenience method for transform(space).scale()
Example
>>> node = createNode("transform") >>> node["scaleX"] = 5.0 >>> node.scale().x 5.0
-
setLimit
(typ, value)¶
-
set_limit
(typ, value)¶
-
shape
(type=None)¶
-
shapes
(type=None, query=None)¶
-
shortestPath
()¶ Return shortest unique path to node
Example
>>> _ = cmds.file(new=True, force=True) >>> parent = createNode("transform", name="myParent") >>> child = createNode("transform", name="myChild", parent=parent) >>> child.shortestPath() == "myChild" True >>> child = createNode("transform", name="myChild") >>> # Now `myChild` could refer to more than a single node >>> child.shortestPath() == '|myChild' True
-
shortest_path
()¶ Return shortest unique path to node
Example
>>> _ = cmds.file(new=True, force=True) >>> parent = createNode("transform", name="myParent") >>> child = createNode("transform", name="myChild", parent=parent) >>> child.shortestPath() == "myChild" True >>> child = createNode("transform", name="myChild") >>> # Now `myChild` could refer to more than a single node >>> child.shortestPath() == '|myChild' True
-
show
()¶ Set visibility to True
-
sibling
(type=None, filter=None)¶
-
siblings
(type=None, filter=110)¶
-
transform
(space=2, time=None)¶ Return TransformationMatrix
-
transformation
()¶
-
translation
(space=2, time=None)¶ Convenience method for transform(space).translation()
Example
>>> node = createNode("transform") >>> node["translateX"] = 5.0 >>> node.translation().x 5.0
-
-
class
cmdx.
Plug
(node, mplug, unit=None, key=None)¶ -
animate
(values, tangents=None)¶ Treat values as time:value pairs and animate this attribute
Example
>>> _ = cmds.file(new=True, force=True) >>> node = createNode("transform") >>> node["tx"] = {1: 0.0, 5: 1.0, 10: 0.0} >>> node["rx"] = {1: 0.0, 5: 1.0, 10: 0.0} >>> node["sx"] = {1: 0.0, 5: 1.0, 10: 0.0} >>> node["v"] = {1: True, 5: False, 10: True}
# Direct function call >>> node[“ry”].animate({1: 0.0, 5: 1.0, 10: 0.0})
# Interpolation >>> node[“rz”].animate({1: 0.0, 5: 1.0, 10: 0.0}, Smooth)
-
animated
(recursive=True)¶ Return whether this attribute is connected to an animCurve
Parameters: recursive (bool, optional) – Should I travel to connected attributes in search of an animCurve, or only look to the immediate connection?
-
append
(value, autofill=False)¶ Add value to end of self, which is an array
Parameters: - value (object) – If value, create a new entry and append it. If cmdx.Plug, create a new entry and connect it.
- autofill (bool) – Append to the next available index. This performs a search for the first unconnected value of an array to reuse potentially disconnected plugs and optimise space.
Example
>>> _ = cmds.file(new=True, force=True) >>> node = createNode("transform", name="appendTest") >>> node["myArray"] = Double(array=True) >>> node["myArray"].append(1.0) >>> node["notArray"] = Double() >>> node["notArray"].append(2.0) Traceback (most recent call last): ... TypeError: "|appendTest.notArray" was not an array attribute >>> node["myArray"][0] << node["tx"] >>> node["myArray"][1] << node["ty"] >>> node["myArray"][2] << node["tz"] >>> node["myArray"].count() 3 >>> # Disconnect doesn't change count >>> node["myArray"][1].disconnect() >>> node["myArray"].count() 3 >>> node["myArray"].append(node["ty"]) >>> node["myArray"].count() 4 >>> # Reuse disconnected slot with autofill=True >>> node["myArray"].append(node["rx"], autofill=True) >>> node["myArray"].count() 4
-
arrayIndices
¶
-
array_indices
¶
-
asDouble
(time=None)¶ Return plug as double (Python float)
Example
>>> node = createNode("transform") >>> node["translateX"] = 5.0 >>> node["translateX"].asDouble() 5.0
-
asEuler
(order=0, time=None)¶
-
asEulerRotation
(order=0, time=None)¶
-
asMatrix
(time=None)¶ Return plug as MatrixType
Example
>>> node1 = createNode("transform") >>> node2 = createNode("transform", parent=node1) >>> node1["translate"] = (0, 5, 0) >>> node2["translate"] = (0, 5, 0) >>> plug1 = node1["matrix"] >>> plug2 = node2["worldMatrix"][0] >>> mat1 = plug1.asMatrix() >>> mat2 = plug2.asMatrix() >>> mat = mat1 * mat2 >>> tm = TransformationMatrix(mat) >>> list(tm.translation()) [0.0, 15.0, 0.0]
-
asPoint
(time=None)¶
-
asQuaternion
(time=None)¶
-
asTime
(time=None)¶
-
asTm
(time=None)¶ Return plug as TransformationMatrix
Example
>>> node = createNode("transform") >>> node["translateY"] = 12 >>> node["rotate"] = 1 >>> tm = node["matrix"].asTransform() >>> [round(v, 1) for v in tm.rotation()] [1.0, 1.0, 1.0] >>> list(tm.translation()) [0.0, 12.0, 0.0]
-
asTransform
(time=None)¶ Return plug as TransformationMatrix
Example
>>> node = createNode("transform") >>> node["translateY"] = 12 >>> node["rotate"] = 1 >>> tm = node["matrix"].asTransform() >>> [round(v, 1) for v in tm.rotation()] [1.0, 1.0, 1.0] >>> list(tm.translation()) [0.0, 12.0, 0.0]
-
asTransformationMatrix
(time=None)¶ Return plug as TransformationMatrix
Example
>>> node = createNode("transform") >>> node["translateY"] = 12 >>> node["rotate"] = 1 >>> tm = node["matrix"].asTransform() >>> [round(v, 1) for v in tm.rotation()] [1.0, 1.0, 1.0] >>> list(tm.translation()) [0.0, 12.0, 0.0]
-
asVector
(time=None)¶
-
as_double
(time=None)¶ Return plug as double (Python float)
Example
>>> node = createNode("transform") >>> node["translateX"] = 5.0 >>> node["translateX"].asDouble() 5.0
-
as_euler
(order=0, time=None)¶
-
as_euler_rotation
(order=0, time=None)¶
-
as_matrix
(time=None)¶ Return plug as MatrixType
Example
>>> node1 = createNode("transform") >>> node2 = createNode("transform", parent=node1) >>> node1["translate"] = (0, 5, 0) >>> node2["translate"] = (0, 5, 0) >>> plug1 = node1["matrix"] >>> plug2 = node2["worldMatrix"][0] >>> mat1 = plug1.asMatrix() >>> mat2 = plug2.asMatrix() >>> mat = mat1 * mat2 >>> tm = TransformationMatrix(mat) >>> list(tm.translation()) [0.0, 15.0, 0.0]
-
as_point
(time=None)¶
-
as_quaternion
(time=None)¶
-
as_time
(time=None)¶
-
as_transform
(time=None)¶ Return plug as TransformationMatrix
Example
>>> node = createNode("transform") >>> node["translateY"] = 12 >>> node["rotate"] = 1 >>> tm = node["matrix"].asTransform() >>> [round(v, 1) for v in tm.rotation()] [1.0, 1.0, 1.0] >>> list(tm.translation()) [0.0, 12.0, 0.0]
-
as_transformation_matrix
(time=None)¶ Return plug as TransformationMatrix
Example
>>> node = createNode("transform") >>> node["translateY"] = 12 >>> node["rotate"] = 1 >>> tm = node["matrix"].asTransform() >>> [round(v, 1) for v in tm.rotation()] [1.0, 1.0, 1.0] >>> list(tm.translation()) [0.0, 12.0, 0.0]
-
as_vector
(time=None)¶
-
attribute
()¶ Return the attribute MObject of this cmdx.Plug
-
channelBox
¶ Is the attribute visible in the Channel Box?
-
channel_box
¶ Is the attribute visible in the Channel Box?
-
clone
(name, shortName=None, niceName=None)¶ Return AbstractAttribute of this exact plug
This can be used to replicate one plug on another node, including default values, min/max values, hidden and keyable states etc.
Examples
>>> parent = createNode("transform") >>> cam = createNode("camera", parent=parent) >>> original = cam["focalLength"] >>> clone = original.clone("focalClone")
# Original setup is preserved >>> clone[“min”] 2.5 >>> clone[“max”] 100000.0
>>> cam.addAttr(clone) >>> cam["focalClone"].read() 35.0
# Also works with modifiers >>> with DagModifier() as mod: … clone = cam[“fStop”].clone(“fClone”) … _ = mod.addAttr(cam, clone) … >>> cam[“fClone”].read() 5.6
-
connect
(other, force=True)¶
-
connected
¶ Return whether or not this attribute has an input connection
Examples
>>> node = createNode("transform") >>> node["tx"] >> node["ty"]
# ty is connected, tx is not >>> node[“tx”].connected False
>>> node["ty"].connected True
-
connection
(type=None, source=True, destination=True, plug=False, unit=None)¶ Return first connection from
connections()
-
connections
(type=None, source=True, destination=True, plugs=False, unit=None)¶ Yield plugs connected to self
Parameters: - type (int, optional) – Only return nodes of this type
- source (bool, optional) – Return source plugs, default is True
- destination (bool, optional) – Return destination plugs, default is True
- plugs (bool, optional) – Return connected plugs instead of nodes
- unit (int, optional) – Return plug in this unit, e.g. Meters
Example
>>> _ = cmds.file(new=True, force=True) >>> a = createNode("transform", name="A") >>> b = createNode("multDoubleLinear", name="B") >>> a["ihi"] << b["ihi"] >>> a["ihi"].connection() == b True >>> b["ihi"].connection() == a True >>> a["ihi"] 2 >>> b["arrayAttr"] = Long(array=True) >>> b["arrayAttr"][0] >> a["ihi"] >>> b["arrayAttr"][1] >> a["visibility"] >>> a["ihi"].connection() == b True >>> a["ihi"].connection(plug=True) == b["arrayAttr"][0] True >>> a["visibility"].connection(plug=True) == b["arrayAttr"][1] True >>> b["arrayAttr"][1].connection(plug=True) == a["visibility"] True
-
count
()¶
-
default
¶ Return default value of plug
-
disconnect
(other=None, source=True, destination=True)¶ Disconnect self from other
Parameters: other (Plug, optional) – If none is provided, disconnect everything Example
>>> node1 = createNode("transform") >>> node2 = createNode("transform") >>> node2["tx"].connection() is None True >>> node2["ty"].connection() is None True >>> >>> node2["tx"] << node1["tx"] >>> node2["ty"] << node1["ty"] >>> node2["ty"].connection() is None False >>> node2["tx"].connection() is None False >>> >>> node2["tx"].disconnect(node1["tx"]) >>> node2["ty"].disconnect() >>> node2["tx"].connection() is None True >>> node2["ty"].connection() is None True
-
editable
¶
-
extend
(values)¶ Append multiple values to the end of an array
Parameters: values (tuple) – If values, create a new entry and append it. If cmdx.Plug’s, create a new entry and connect it. Example
>>> node = createNode("transform") >>> node["myArray"] = Double(array=True) >>> node["myArray"].extend([1.0, 2.0, 3.0]) >>> node["myArray"][0] 1.0 >>> node["myArray"][-1] 3.0
-
fn
()¶ Return the correct function set for the plug attribute MObject
-
hide
()¶ Hide attribute from channel box
Note: An attribute cannot be hidden from the channel box and keyable at the same time. Therefore, this method also makes the attribute non-keyable.
Supports array and compound attributes too.
-
input
(type=None, plug=False, unit=None)¶ Return input connection from
connections()
-
isArray
¶
-
isCompound
¶
-
keyable
¶ Is the attribute keyable?
-
lock
()¶ Convenience function for plug.locked = True
Examples
# Static attributes work just fine >>> _new() >>> node1 = createNode(“transform”, name=”node1”) >>> node1[“translateX”].locked False >>> node1[“translateX”].locked = True >>> _save() >>> _load() >>> node1 = encode(“node1”) >>> node1[“translateX”].locked True
# Dynamic attributes work too >>> _new() >>> node1 = createNode(“transform”, name=”node1”) >>> node1[“dynamicAttr”] = Double() >>> node1[“dynamicAttr”].locked False >>> node1[“dynamicAttr”].locked = True >>> _save() >>> _load() >>> node1 = encode(“node1”) >>> node1[“dynamicAttr”].locked True
-
lockAndHide
()¶
-
lock_and_hide
()¶
-
locked
¶
-
name
(long=True, full=False)¶ Return name part of an attribute
Examples
>>> persp = encode("persp") >>> persp["translateX"].name() 'translateX' >>> persp["tx"].name() 'translateX' >>> persp["tx"].name(long=False) 'tx' >>> persp["tx"].name(full=True) 'translate.translateX' >>> persp["tx"].name(long=False, full=True) 't.tx' >>> persp["worldMatrix"].name() 'worldMatrix' >>> persp["worldMatrix"][0].name() 'worldMatrix[0]'
-
nextAvailableIndex
(startIndex=0)¶ Find the next unconnected element in an array plug
Array plugs have both a “logical” and “physical” index. Accessing any array plug via index means to access it’s logical element.
[0]<—- plug1 [.] [.] [3]<—- plug2 [4]<—- plug3 [5]<—- plug4 [.] [7]<—- plug5
In the above scenario, 5 plugs are connected by 5 physical indices, and yet the last index is 7. 7 is a logical index.
This function walks each element from the startIndex in search of the first available index, in this case [1].
Examples
>>> transform = createNode("transform") >>> mult = createNode("multMatrix") >>> mult["matrixIn"][0] << transform["matrix"] >>> mult["matrixIn"][1] << transform["matrix"] >>> mult["matrixIn"][2] << transform["matrix"]
# 3 logical indices are occupied >>> mult[“matrixIn”].count() 3
# Disconnecting affects the physical count >>> mult[“matrixIn”][1].disconnect() >>> mult[“matrixIn”].count() 2
# But the last logical index is still 2 >>> mult[“matrixIn”].arrayIndices [0, 2]
# Finally, let’s find the next available index >>> mult[“matrixIn”].nextAvailableIndex() 1
-
next_available_index
(startIndex=0)¶ Find the next unconnected element in an array plug
Array plugs have both a “logical” and “physical” index. Accessing any array plug via index means to access it’s logical element.
[0]<—- plug1 [.] [.] [3]<—- plug2 [4]<—- plug3 [5]<—- plug4 [.] [7]<—- plug5
In the above scenario, 5 plugs are connected by 5 physical indices, and yet the last index is 7. 7 is a logical index.
This function walks each element from the startIndex in search of the first available index, in this case [1].
Examples
>>> transform = createNode("transform") >>> mult = createNode("multMatrix") >>> mult["matrixIn"][0] << transform["matrix"] >>> mult["matrixIn"][1] << transform["matrix"] >>> mult["matrixIn"][2] << transform["matrix"]
# 3 logical indices are occupied >>> mult[“matrixIn”].count() 3
# Disconnecting affects the physical count >>> mult[“matrixIn”][1].disconnect() >>> mult[“matrixIn”].count() 2
# But the last logical index is still 2 >>> mult[“matrixIn”].arrayIndices [0, 2]
# Finally, let’s find the next available index >>> mult[“matrixIn”].nextAvailableIndex() 1
-
niceName
¶ The nice name of this plug, visible in e.g. Channel Box
Examples
>>> _new() >>> node = createNode("transform", name="myTransform")
# Return pairs of nice names for compound attributes >>> node[“scale”].niceName == (“Scale X”, “Scale Y”, “Scale Z”) True
>>> assert node["translateY"].niceName == "Translate Y" >>> node["translateY"].niceName = "New Name" >>> assert node["translateY"].niceName == "New Name"
# The nice name is preserved on scene open >>> _save() >>> _load() >>> node = encode(“myTransform”) >>> assert node[“translateY”].niceName == “New Name”
-
node
()¶
-
output
(type=None, plug=False, unit=None)¶ Return first output connection from
connections()
-
outputs
(type=None, plugs=False, unit=None)¶ Return output connections from
connections()
-
path
(full=False)¶ Return path to attribute, including node path
Examples
>>> persp = encode("persp") >>> persp["translate"].path() '|persp.translate' >>> persp["translateX"].path() '|persp.translateX' >>> persp["translateX"].path(full=True) '|persp.translate.translateX' >>> persp["worldMatrix"].path() '|persp.worldMatrix' >>> persp["worldMatrix"][0].path() '|persp.worldMatrix[0]'
-
plug
()¶ Return the MPlug of this cmdx.Plug
-
pull
()¶ Pull on a plug, without serialising any value. For performance.
-
read
(unit=None, time=None)¶ Read attribute value
Parameters: - unit (int, optional) – Unit with which to read plug
- time (float, optional) – Time at which to read plug
Example
>>> node = createNode("transform") >>> node["ty"] = 100.0 >>> node["ty"].read() 100.0 >>> node["ty"].read(unit=Meters) 1.0
-
reset
()¶ Restore plug to default value
-
show
()¶ Show attribute in channel box
Note: An attribute can be both visible in the channel box and non-keyable, therefore, unlike
hide()
, this method does not alter the keyable state of the attribute.
-
source
(unit=None)¶
-
type
()¶ Retrieve API type of plug as string
Example
>>> node = createNode("transform") >>> node["translate"].type() 'kAttribute3Double' >>> node["translateX"].type() 'kDoubleLinearAttribute'
-
typeClass
()¶ Retrieve cmdx Attribute class of plug, e.g. Double
This reverse-engineers the plug attribute into the attribute class used to originally create it. It’ll work on attributes not created with cmdx as well, but won’t catch’em’all (see below)
Examples
>>> victim = createNode("transform") >>> victim["enumAttr"] = Enum() >>> victim["stringAttr"] = String() >>> victim["messageAttr"] = Message() >>> victim["matrixAttr"] = Matrix() >>> victim["longAttr"] = Long() >>> victim["doubleAttr"] = Double() >>> victim["floatAttr"] = Float() >>> victim["double3Attr"] = Double3() >>> victim["booleanAttr"] = Boolean() >>> victim["angleAttr"] = Angle() >>> victim["timeAttr"] = Time() >>> victim["distanceAttr"] = Distance() >>> victim["compoundAttr"] = Compound(children=[Double("Temp")])
>>> assert victim["enumAttr"].typeClass() == Enum >>> assert victim["stringAttr"].typeClass() == String >>> assert victim["messageAttr"].typeClass() == Message >>> assert victim["matrixAttr"].typeClass() == Matrix >>> assert victim["longAttr"].typeClass() == Long >>> assert victim["doubleAttr"].typeClass() == Double >>> assert victim["floatAttr"].typeClass() == Float >>> assert victim["double3Attr"].typeClass() == Double3 >>> assert victim["booleanAttr"].typeClass() == Boolean >>> assert victim["angleAttr"].typeClass() == Angle >>> assert victim["timeAttr"].typeClass() == Time >>> assert victim["distanceAttr"].typeClass() == Distance >>> assert victim["compoundAttr"].typeClass() == Compound
# Unsupported # >>> victim[“dividerAttr”] = Divider() # >>> victim[“double2Attr”] = Double2() # >>> victim[“double4Attr”] = Double4() # >>> victim[“angle2Attr”] = Angle2() # >>> victim[“angle3Attr”] = Angle3() # >>> victim[“distance2Attr”] = Distance2() # >>> victim[“distance3Attr”] = Distance3() # >>> victim[“distance4Attr”] = Distance4()
-
type_class
()¶ Retrieve cmdx Attribute class of plug, e.g. Double
This reverse-engineers the plug attribute into the attribute class used to originally create it. It’ll work on attributes not created with cmdx as well, but won’t catch’em’all (see below)
Examples
>>> victim = createNode("transform") >>> victim["enumAttr"] = Enum() >>> victim["stringAttr"] = String() >>> victim["messageAttr"] = Message() >>> victim["matrixAttr"] = Matrix() >>> victim["longAttr"] = Long() >>> victim["doubleAttr"] = Double() >>> victim["floatAttr"] = Float() >>> victim["double3Attr"] = Double3() >>> victim["booleanAttr"] = Boolean() >>> victim["angleAttr"] = Angle() >>> victim["timeAttr"] = Time() >>> victim["distanceAttr"] = Distance() >>> victim["compoundAttr"] = Compound(children=[Double("Temp")])
>>> assert victim["enumAttr"].typeClass() == Enum >>> assert victim["stringAttr"].typeClass() == String >>> assert victim["messageAttr"].typeClass() == Message >>> assert victim["matrixAttr"].typeClass() == Matrix >>> assert victim["longAttr"].typeClass() == Long >>> assert victim["doubleAttr"].typeClass() == Double >>> assert victim["floatAttr"].typeClass() == Float >>> assert victim["double3Attr"].typeClass() == Double3 >>> assert victim["booleanAttr"].typeClass() == Boolean >>> assert victim["angleAttr"].typeClass() == Angle >>> assert victim["timeAttr"].typeClass() == Time >>> assert victim["distanceAttr"].typeClass() == Distance >>> assert victim["compoundAttr"].typeClass() == Compound
# Unsupported # >>> victim[“dividerAttr”] = Divider() # >>> victim[“double2Attr”] = Double2() # >>> victim[“double4Attr”] = Double4() # >>> victim[“angle2Attr”] = Angle2() # >>> victim[“angle3Attr”] = Angle3() # >>> victim[“distance2Attr”] = Distance2() # >>> victim[“distance3Attr”] = Distance3() # >>> victim[“distance4Attr”] = Distance4()
-
unlock
()¶ Convenience function for plug.locked = False
-
writable
¶ Can the user write to this attribute?
Convenience for combined call to plug.connected and plug.locked.
Example
>> if node[“translateX”].writable: .. node[“translateX”] = 5
-
write
(value)¶
-
Attributes¶
-
class
cmdx.
Enum
(name, fields=None, default=0, label=None, **kwargs)¶
-
class
cmdx.
Divider
(label, **kwargs)¶ Visual divider in channel box
-
class
cmdx.
String
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Message
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Matrix
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Long
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Double
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Double3
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Boolean
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
AbstractUnit
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Angle
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Time
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
-
class
cmdx.
Distance
(name, default=None, label=None, shortName=None, writable=None, readable=None, cached=None, storable=None, keyable=None, hidden=None, min=None, max=None, channelBox=None, affectsAppearance=None, affectsWorldSpace=None, array=False, indexMatters=None, connectable=True, disconnectBehavior=2, help=None)¶
Units¶
-
cmdx.
Degrees
= 2¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Radians
= 1¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
AngularMinutes
= 3¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
AngularSeconds
= 4¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Millimeters
= 5¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Centimeters
= 6¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Meters
= 8¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Kilometers
= 7¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Inches
= 1¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Feet
= 2¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Miles
= 4¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
-
cmdx.
Yards
= 3¶ A Maya unit, for unit-attributes such as Angle and Distance
Because the resulting classes are subclasses of int, there is virtually no run-time performance penalty to using it as an integer. No additional Python is called, most notably when passing the integer class to the Maya C++ binding (which wouldn’t call our overridden methods anyway).
The added overhead to import time is neglible.
Interoperability¶
-
cmdx.
encode
(path)¶ Convert relative or absolute path to cmdx Node
Fastest conversion from absolute path to Node
Parameters: path (str) – Absolute or relative path to DAG or DG node
-
cmdx.
decode
(node)¶ Convert cmdx Node to shortest unique path
This is the same as node.shortestPath() To get an absolute path, use node.path()